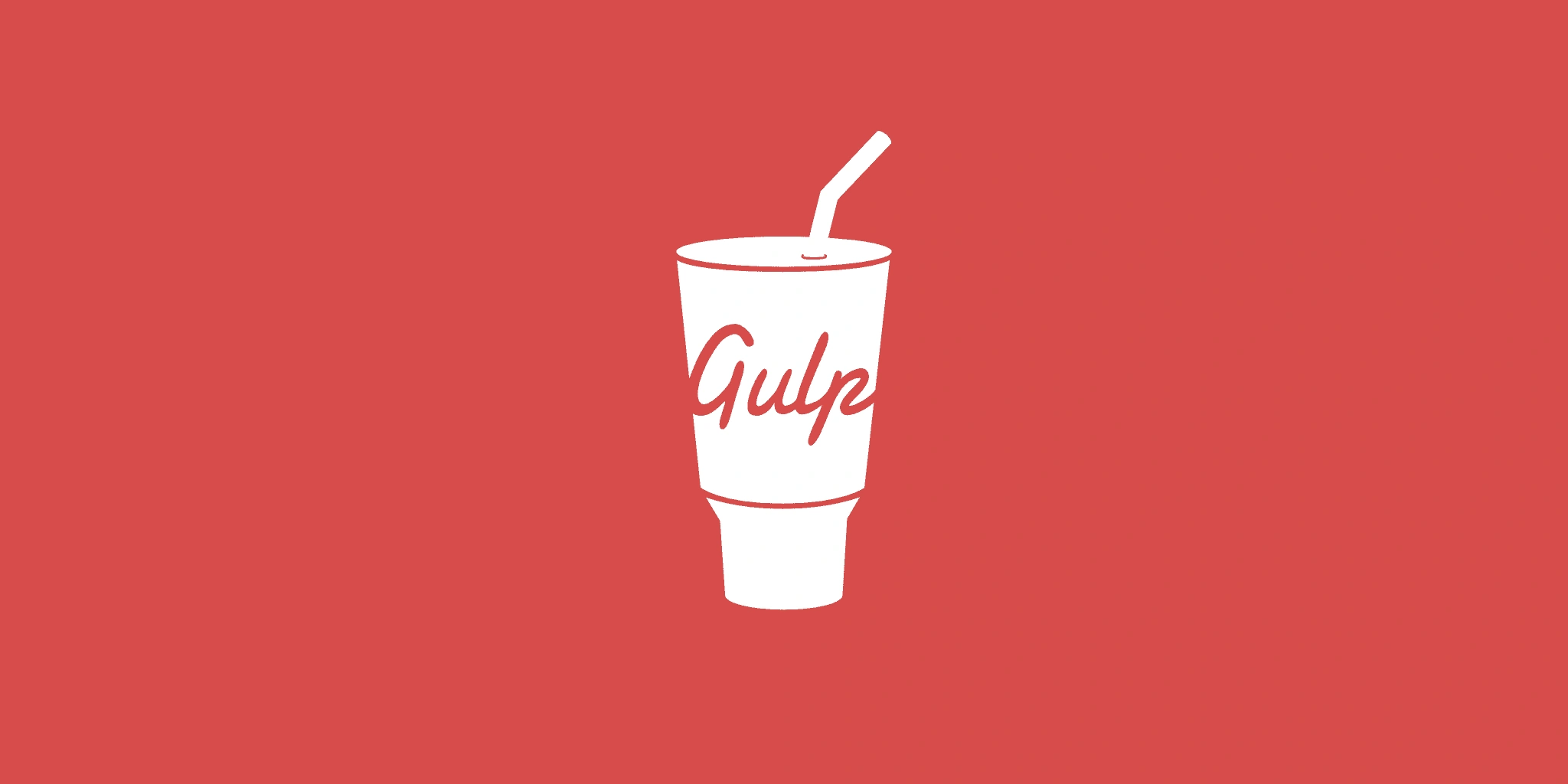
Why Gulp is Awesome
I realised the other day that I had a project that contained 100’s of unminified JS files and decided to look for the most efficient way of automagically minifying them so that a) they are harder to read/unpick and b) the site will run faster; well web-application in this case.
I consulted the forums and got a tip to use ‘Gulp’.
If you’ve never used it then I really recommend that you try it out; it will well and truly blow your mind. I am now wondering how I have lived for so long without it and you will too.
With gulp, I created one file and all of this minification just magically happened. Admittedly, it took a little bit of trial and error to get right but now that I’ve done it I can use it over and over again (and in other projects).
I’ll show you the file that I have a bit later on, but right now I’m sure you’re more interested in how to install it.
I’m on a Mac so all of these instructions are relevant to that. If you’re not using a Mac for web development then you’ll need to buy a Mac because they are better find other instructions.
One
Make sure node is installed on your computer by running in your terminal node -v
. If it’s not installed then you’ll need to. More info can be found on the nodejs website. You might find this complete nodejs installation tutorial useful too.
Two
With node installed, run npm install –global gulp
. You’ll probably need to add a sudo
infront of that too.
Three
Now that node is installed globally, use terminal to navigate to your project directory. Something like this should work:
cd ~/Sites/project/htdocs/
(Assuming your project is stored inside an ‘htdocs’ folder, inside a ‘project’ folder inside a ‘Sites’ folder)
Four
Run sudo npm install gulp
. This will give you barebones gulp in your project. We’re going to beautify the JS that we are using and then ugligy/minify js for use on the website. In order to do that we also need to run sudo npm install gulp-beautify
and sudo npm install gulp-uglify
(or sudo npm install gulp-beautify gulp-uglify
if you’re a bit more efficient. We also need the rename feature so we’ll install that too; sudo npm install gulp-rename
. The next step outlines the full code you need to do that.
Five
var gulp = require('gulp'),
rename = require('gulp-rename'),
beautify = require('gulp-beautify'),
uglify = require('gulp-uglify');
gulp.task('beautify', function(){
var stream = gulp.src(['js/**/*.js','!js/**/*.max.js'])
.pipe(rename({suffix:'.max'}))
.pipe(beautify())
.pipe(gulp.dest('js'));
return stream;
});
gulp.task('uglify', ['beautify'], function(){
var stream = gulp.src(['js/**/*.max.js'])
.pipe(uglify())
.pipe(rename(function(path) {
path.basename = path.basename.replace('.max','');
}))
.pipe(gulp.dest('js'));
return stream;
});
gulp.task('default', ['uglify']);
UH, whaaaaat?
Okay, the code above might look at little bit confusing to begin with, but stick with it… here’s an explanation:
First, we declare variables that we’ll use in our script… the first one is absolutely needed for everything; ‘gulp’. After that we declare the other variables that we are going to use for this script.
From then onwards it’s probably easiest to read from bottom to top.
gulp.task('default', ['uglify']);
This says "declare the default gulp task as ‘uglify’"
gulp.task('uglify', ['beautify'], function(){
var stream = gulp.src(['js/**/*.max.js'])
.pipe(uglify())
.pipe(rename(function(path) {
path.basename = path.basename.replace('.max','');
}))
.pipe(gulp.dest('js'));
return stream;
});
This reads as ‘make a new task called uglify that cannot run until beautify has finished’. This task then does the following: Goes and grabs all of the files that end in .max.js from within the /js/ folder (the extra stars in there allow it to search for files nested inside of folders that are inside the /js/ folder). It then uglifys those JS files, renames them by removing the ‘.max’ from the filename and then saves them back in the same location that they came from.
Of course, none of that can work until the beautify block has run.
gulp.task('beautify', function(){
var stream = gulp.src(['js/**/*.js','!js/**/*.max.js'])
.pipe(rename({suffix:'.max'}))
.pipe(beautify())
.pipe(gulp.dest('js'));
return stream;
});
This reads as ‘make a new task called beautify’. This task then does the following: Goes and grabs all of the files that end in .js (but not ‘.max.js’) from within the /js/ folder. It then renames those JS files with ‘.max.js’ extensions, beautifies them and saves them.
Six
In your terminal run gulp
This will run your script (because we named the task ‘default’) and do all of the magic for you.
TL;DR?
Use the script in Step 5 to save all of your JS files as beautified files and minified files. The minified files will be saved with ‘.js’ extensions and the beautified with ‘.max.js’ extensions. From then onwards, you use the max.js versions to do your edits and after running the code in Step 6, you’ll have minified files ready for upload.
There’s tons more that Gulp can do, this is barely scratching the surface. I really recommend that you start playing with it! More info can be found on the offical site, here: http://gulpjs.com/